From JavaScript to Scala
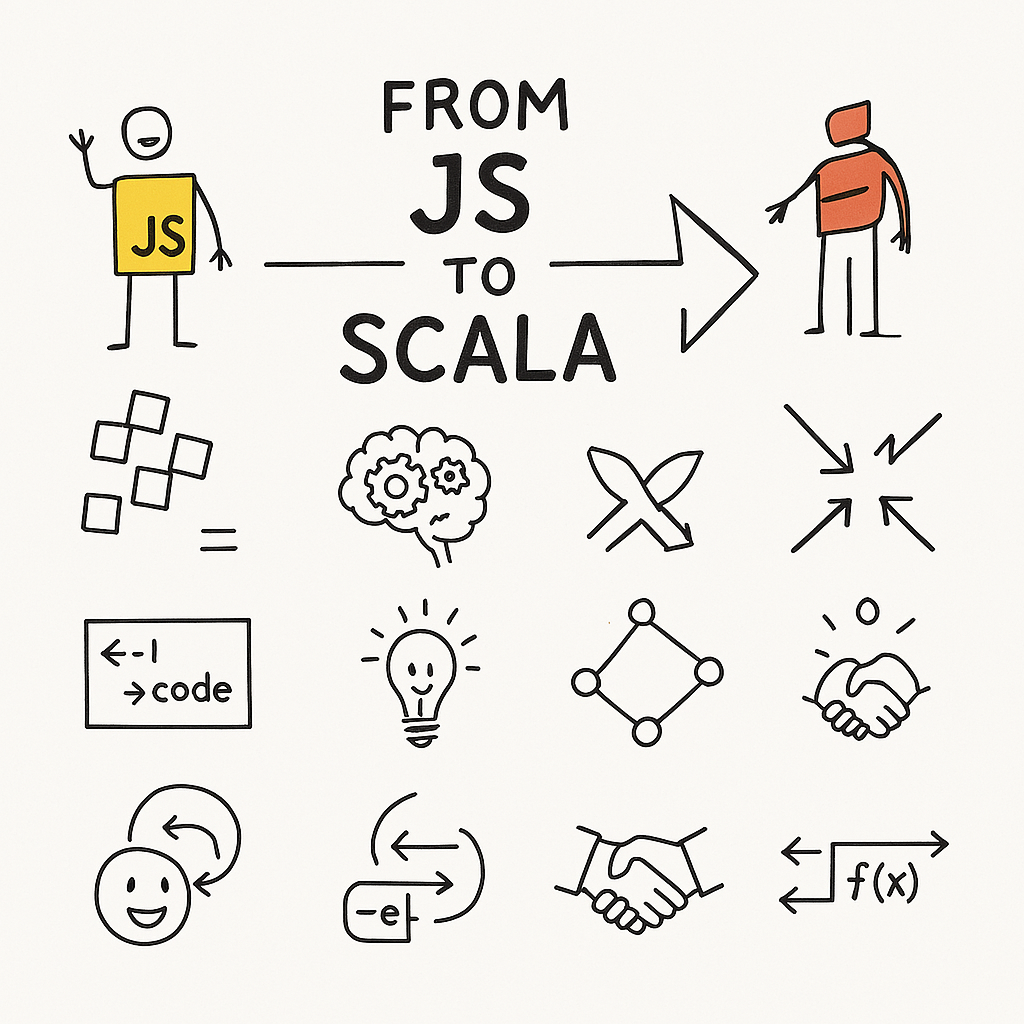
I was writing C# when Node.js came along. You could run JavaScript on the server now. I was in awe. It wasn’t perfect. But it was freeing and dynamic. I went all in.
Then came React. Its mental model aligned perfectly with mine. It was simply the V in MVC. Declarative and flexible. It just made sense. Those were good times. I felt like I was standing at the beginning and could clearly see the future. I went all in, again.
As JavaScript evolved it initially went where I was hoping it would, but then it blew past to... something else? We seemed to lose our way with error handling. We shoved React SSR Frameworks down everyones throat. & we let TypeScript(a superset of javascript) win over Javascript and now it’s everywhere, too a fault.
How do I know? Well when all of my non JS colleagues keep referring to node or javascript as Typescript... that means it's over.
There are bits about Typescript I really like. I like being able to eliminate statically typed defects. No more guessing at what the data
arg is. That has been pretty damn sweet. But everything else? Just tends to get in the way...
Pure ESM, while arguably a better approach to modules adds to the hard edges.
I've tried ejecting from TypeScript and going back to plain JavaScript and pure ESM. But you quickly hit walls trying to tap into the JS ecosystem. Modern JS tooling seems overly dependent on it. You end up having to roll your own everything if you want to eject. You'll eventually find yourself coming back to TypeScript because sadly it is now the least path of resistance.
I'm hopeful for the future of JavaScriptTypescript, but at present it doesn't quite spark joy for me.
I started looking elsewhere. I picked up Golang. Bought the books. Built the apps. I liked it. And it worked rather well. Cross platform binaries out of the box was the chefs kiss. Maybe Go was it?
Maybe not... I really liked it, but it felt too simple, if that even makes sense. I recognize 'simple' is arguably a good thing. But I wanted more depth. Something that could grow with me. Go felt better, but it didn’t make me want to build just for the sake of building again.
Then, over a year ago, I joined AKA Identity and had to jump into Scala. The architect chose it for AKA's data pipeline. I’d never given Scala much thought before. So at first, I had low expectations. Scala seemed to have a reputation, "Something something about functional purists". I pictured obscure, obfuscated one-liners.
Sieve of Eratosthenes(n: Int) => (2 to n) |> (r => r.foldLeft(r.toSet)((ps, x) => if (ps(x)) ps -- (x * x to n by x) else ps))
source: 10 scala one liners to impress your friends
As part of my team's book club, we picked up Oderskys' Programming in Scala. I couldn’t put it down. I ended up getting more books. Along the way, I felt excited again. Scala could be powerful without being heavy handed. Expressive without being verbose. I could apply it creatively. Artfully. But only, if I wanted too... Scala for the most part, could be pragmatic and simple.
& I really appreciated that the Scala devs at AKA leaned into writing pragmatic Scala. Had they not, it's entirely possible I would not have ever given Scala a fair shake.
My time so far with Scala, reminds me of those early days with Node. The urge to build, creatively, outside of the box. It’s hard to explain. But it felt good. As I learned from Oderskys' writing and others, my mind adapted to Scala’s mental models. It was like the Tetris pieces were falling into place, at the right angle, and clicking. To say it was satisfying would be an understatement.
So now, I’m thinking about writing this series; From JS to Scala.
If you're a fellow js dev and feel like I did, largely stuck and and a bit unsatisfied, looking for creative freedom. Maybe Scala will do it for you, too.
Fwiw, I am not making a 'die hard' switch to Scala. I'll continue to write in TypeScript, Go, and other languages. Scala hasn't replaced them, it made me better at using them. It opened up my mental model and changed how I think about the problem at hand. It made me a more thoughtful coder.
Heres what I am thinking so far:
- Async, Multithreading, Coroutines: As They Should Be
- Compare how Scala and Node.js handle concurrency and CPU-bound tasks.
- Why async in Node.js trips up developers, who inadvertently block the main thread.
- How this compares to Async in Scala: Native multithreading and coroutine support mean true parallelism without blocking the main thread.
- Types: Practical and Reliable Typing Without Verbosity/Gymnastics
- Why Scala’s type system feels more natural, less verbose, and more flexible compared to TypeScript.
- Using case classes and sealed traits for structured, type-safe data.
- How type inference reduces boilerplate without sacrificing clarity.
- Compare a simple(at least it should be) implementation of returning [Error, GenericType] in TypeScript vs Scala.
- For-Comprehensions: Concise Async Control Flow
- Use for-comprehensions for asynchronous operations and data transformation.
- Replace complex callback chains or async/await patterns without reducing all errors into a single try-catch channel like JavaScript does.
- Real-world examples comparing JS async/await and promise chains to Scala’s for-comprehensions.
- Pattern Matching for Control Flow
- How Scala’s pattern matching offers a more intuitive way to manage control flow.
- Demonstrate pattern matching with both simple and complex data types.
- Show how pattern matching can cleanly handle data processing and branching logic compared to typical control flow you'd see in Javascript.
- Options and the None Type: No More Null & Undefined Shenanigans
- Compare Scala’s Options approach to TypeScript’s nullable types.
- Highlight the confusion between valid JavaScript and TypeScript's rigidity, where making valid JS work freely often results in weird and inconsistent handling of null, undefined, and other falsey values.
- & How ultimately, Scala’s Option makes handling potential value absence straightforward, eliminating null and undefined entirely.
- Writing APIs That Feel Native
- Imagine being able to define a custom operator in TypeScript, like Akka’s
!
operator in Scala, to send messages with a simple syntax:myService ! "Hello, World!"
. - In TypeScript, achieving this would require writing a custom compiler plugin, and even then, it would be cumbersome to adopt and maintain. In Scala, the ability to express syntax like this is built in. It enables creative expression, to solve the problem at hand.
- Imagine being able to define a custom operator in TypeScript, like Akka’s
- Pragmatic Scalability: Avoiding Clever Code
- This will show that Scala can be written using simple and pragmatic semantics. Which is important for new comers like myself.
- Discusses the reality that Scala can also enable very obscure, albeit clever, code. & as Odersky says, we should apply this artfully.
- Highlight libraries that promote pragmatic Scala usage, like those by Li Haoyi (e.g., lihaoyi’s libraries).
- Practical examples of balancing expressiveness with readability.
- Building and Deploying a Scala App: From Nuts to Soup
- A full example of building, testing, and deploying a Scala application. It can be a lot easier than you'd think.
& I am sure plenty more after I groom my past notes from the books I've read.
** Topic Ideas From Feedback: